Документация API
Пошаговый план базовой интеграции
- Зарегистрируйте аккаунт в CryptoScan.
Добавьте новый Мерчант.
- Название — название сайта. Это название будут видеть в платежном виджете.
- URL Мерчанта — ссылка на сайт.
- Логотип — будет отображен в платежном виджите.
Кто платит комиссию сервиса — кто будет оплачивать комиссию CryptoScan: покупатель или Вы.
Максимальный разряд дроби — ограничивает максимальный размер дробной части числа.
Пример чисел для значения 3:
1.234
,0.789
,2.345
.Пример чисел для значения 5:
1.23456
,0.98765
,2.34567
.Кошелёк USDT TRC20 — можете воспользоваться для его создания TronLink, TrustWallet или другой счёт.
Ссылка на WebHook — URL на Ваш обработчик, куда будут поступить сообщения об успешной или просроченной оплате.
После создания Мерчанта — Вам будут доступны ключи для работы с API.
Выберите тип аутентификации: по приватном ключу или по подписи.
- Напишите функционал создания платежа в своём приложении. Можете воспользоваться нашим решением для взаимодействия с API. Для создания платежа с нашим виджетом — воспользуйтесь методом API «Создание виджета для Инвойса». Если Вы хотите сделать виджет собственный виджет оплаты — воспользуйтесь «Создание Инвойса».
- Напишите функционал обработчика для WebHook-сообщений.
Готовые решения для интеграции
- CryptoScan PHP Client
- Плагин для XenForo — инструкции в README_*.MD
Основные сведения
Термины
- Пользователь — лицо, которое использует действующую систему для выполнения конкретной функции.
- Мерчант — сайт Пользователя, добавленный в разделе Мерчанты.
- Инвойс — созданный Пользователем запрос на отслеживание платежа в сети TRON в рамках конкретного Мерчанта.
- Пользователь API — лицо, которое использует API действующей системы для выполнения конкретной функции.
- Плательщик — лицо, которое должно оплатить созданный для него Инвойс.
Запрос
Базовый URL:
https://cryptoscan.one/api/v1
Тело ответа:
success
- флаг, в зависимости от успешности запроса - содержит true/falsedata
- содержит результат выполнения запроса. При неудачном запросе - возвращает информацию об ошибке
Успешный запрос:
{ "success": true, "data": { "property": "value", "second_property": "second_value" } }
Запросы с ошибкой:
{ "success": false, "data": { "name": "Unauthorized", "message" : "Your request was made with invalid credentials", "code": 0, "status": 401 } }
Данный ответ включает перечень ошибок в свойстве errors
:
{ "success": false, "data": { "message": "Invalid request data", "errors": { "amount": [ "Amount cannot be blank." ], "client_reference_id": [ "Client Reference Id cannot be blank." ] } } }* Параметр
message
может быть не задан
Аутентификация
Есть два вида аутентификации — по приватному ключу или через подпись запроса. Метод аутентификации переключается в настройках.
Для любого типа аутентификации необходимо передавать публичный ключ Мерчанта в заголовке: public-key
.
Аутентификация по приватному ключу
Передайте приватный ключ Мерчанта в HTTP-заголовке private-key
.
Данный способ более простой в интеграции, но менее надежный, поскольку приватный ключ необходимо передавать по Сети.
Аутентификация по подписи
- Сгенерируйте подпись на основе тела запроса и приватного ключа Мерчанта
- Передайте подпись через HTTP-заголовок
signature
Данный способ сложнее в интеграции и тестировании, чем по подписи, но более надежный, поскольку приватный ключ не передаётся по Сети.
(!) Относитесь к приватному ключу как к паролю. Не передавайте его третьим лицам.Генерирование подписи
Подпись используется для аутентификации сообщений WebHook и запросов API при аутентификации по подписи. Для этого используется SHA-256 хеширование.
Для создании подписи необходимо, произвести следующие действия над параметрами запроса:
- Отсортировать по индексу в порядке возрастания
- Закодировать в URL-кодировке
- Хэшировать используя алгоритм SHA-256 HMAC, в качестве секретного ключа — использовать приватный ключ Мерчанта
Пример создания подписи на PHP:
$requestBody['api_key'] = $publicKey; ksort($requestBody);
$signature = hash_hmac('sha256', http_build_query($requestBody), $privateKey);
Чтобы передать подпись в запрос, добавьте её в HTTP-заголовок запроса signature
.
В API запрос также передается публичный ключ, по которому система находит Мерчант. После этого с помощью приватного ключа мерчанта, который устанавливает Пользователь API в разделе мерчантов, система хэширует данные тела запроса создавая аналогичную подпись на своей стороне. Если полученная в API запросе подпись идентична подписи сформированной на стороне сервера, значит Пользователь API прошел аутентификацию.
Формат ответа
Доступные форматы: JSON, XML.
Формат определяется в зависимости от содержимого свойства Accept
в заголовке
запроса.
Формат для JSON:
Accept: application/json
Формат для XML:
Accept: application/xml
Определить ответ вне зависимости от заголовка можно с помощью GET-параметр _format
.
Пример: _format=json
Webhook
Webhook - процедура отправки уведомления на Ваш сервер при наступлении определенного
события с Инвойсом.
После просроченной или успешной оплаты система отправит уведомление на данный URL.
Webhook считается успешно доставленным, если Ваш сервер вернул HTTP-код =
200
.
В случае неуспешной доставки, системой предусмотрены повторные отправки WebHook, которые
отправляются со следующими интервалами:
- Запрос №1: сразу после оплаты
- Запрос №2: 30 секунд
- Запрос №3: 2 минуты
- Запрос №4: 10 минут
- Запрос №5: 60 минут
- Запрос №6: 2 часа
- Запрос №7: 4 часа
- Запрос №8: 6 часов
- Запрос №9: 12 часов
- Запрос №10: 24 часа
После 10-го запроса — новые больше не отправляются. Также после успешной доставки — последующие уведомления не отправляются.
Каждый ответ Вашего сервера на Webhook-сообщение сохраняется, и может быть просмотрен в разделе инвойсов. Указан заголовок ответа и его содержимое обрезанное до 5000 символов.
Основная информация
Метод | POST |
Формат запроса | application/x-www-form-urlencoded; charset=UTF-8 |
Типы сообщений на WebHook:
event_type
—paid
retry_count
— количество попыток повторной отправки сообщения на WebHook-
data
id
— ID платежаwallet
— кошелёк, куда нужно произвести оплатуpayer_wallet
— кошелёк, с которого произведена оплатаtransaction_id
— ID транзакции в сети TRONsource_currency
— Валюта, указанная Пользователем (если была передана валюта не USD)source_amount
— Сумма в валюте пользователя (если была передана валюта не USD)final_amount
— итоговая сумма к оплате (включая "хвост" и комиссию, если её оплачивает покупатель)requested_amount
— сумма к оплате запрошенная пользователемstatus
— статус платежаclient_reference_id
— номер платежа в системе Пользователя API. Должен быть уникальным для каждого Инвойса в рамках Мерчантаmetadata
— произвольная строка, указываемая пользователем при создании платежаcreated_at
— время создания платежа в unixtimepaid_at
— дата обнаружения оплаты в unixtimeexpire_at
— время, когда счёт станет просрочен (помните, мы проверяем платеж только 30 минут после создания)
event_type
—expired
retry_count
— количество попыток повторной отправки сообщения на WebHook-
data
id
— ID платежаwallet
— кошелёк, куда нужно произвести оплатуfinal_amount
— итоговая сумма к оплате (включая "хвост" и комиссию, если её оплачивает покупатель)requested_amount
— сумма к оплате запрошенная пользователемstatus
— статус платежаclient_reference_id
— номер платежа в системе Пользователя API. Должен быть уникальным для каждого Инвойса в рамках Мерчантаmetadata
— произвольная строка, указываемая пользователем при создании платежаcreated_at
— время создания платежа в unixtimeexpire_at
— время, когда счёт станет просрочен (помните, мы проверяем платеж только 30 минут после создания)
event_type
—paid_manually
- возникает при ручном подтверждении инвойсаretry_count
— количество попыток повторной отправки сообщения на WebHook-
data
id
— ID платежаwallet
— кошелёк, куда нужно произвести оплатуfinal_amount
— итоговая сумма к оплате (включая "хвост" и комиссию, если её оплачивает покупатель)requested_amount
— сумма к оплате запрошенная пользователемstatus
— статус платежаclient_reference_id
— номер платежа в системе Пользователя API. Должен быть уникальным для каждого Инвойса в рамках Мерчантаmetadata
— произвольная строка, указываемая пользователем при создании платежаcreated_at
— время создания платежа в unixtimeexpire_at
— время, когда счёт станет просрочен (помните, мы проверяем платеж только 30 минут после создания)
Ручное проведение платежа доступно в лично кабинете пользователя. Для этого:
- перейдите по ссылке
- в списке инвойсов найдите нужный платеж
- нажмите на кнопку "Ручное проведение платежа"
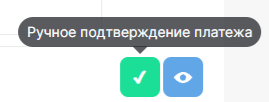
Создание Инвойса
Создаёт запрос на отслеживание транзакции в сети TRON.
(!) Используя этот метод API, Пользователю API необходимо самостоятельно вывести данные для Плательщика: реквизиты, сумму, срок оплаты. Если Вы не хотите делать собственный платежный виджет — воспользуйтесь нашим.
Основная информация
Метод | POST invoice |
Пример URL | https://cryptoscan.one/api/v1/invoice |
Параметры запроса
Имя параметра | Тип данных | Обязательно | Описание |
---|---|---|---|
amount | float | + | Сумма к оплате |
currency | string | Валюта суммы к оплате, которую указал Пользователь. Если этот параметр не задан, по умолчанию считается, что сумма в долларах США. Узнать доступные валюты и их курсы вы можете в разделе Валютный курс. | |
client_reference_id | string | + | Номер платежа в системе Пользователя API. Должен быть уникальным для каждого Инвойса в рамках Мерчанта. |
metadata | string | Произвольная строка до 2000 символов |
Параметры ответа:
Возвращает |
Объект инвойса:
|
Пример ответа:
{ "success": true, "data": { "id": "12589", "final_amount": "125.69", "wallet": "TNVq3iEcaGWbbsR34MTdg1JMTxvYFU8Qir", "expire_at": "1677422490", } }
Создание Виджета для Инвойса
Кроме создание инвойса на оплату, данный запрос создает страницу с виджетом и возвращает URL на неё. Перейдя на эту страницу, Плательщик сможет произвести оплату.
Пример виджета можно посмотреть на странице
Основная информация
Метод | POST invoice/widget |
Пример URL | https://cryptoscan.one/api/v1/invoice/widget |
Параметры запроса
Имя параметра | Тип данных | Обязательно | Описание |
---|---|---|---|
amount | float | + | Сумма платежа |
currency | string | Валюта суммы платежа, которую указал Пользователь. Если этот параметр не задан, по умолчанию считается, что сумма в долларах США. | |
client_reference_id | string | + | Номер платежа в системе Пользователя API. Должен быть уникальным для каждого Инвойса в рамках Мерчанта. |
widget_description | string | Описание, которое увидит Плательщик на странице оплаты до 100 символов | |
metadata | string | Произвольная строка, указываемая пользователем при создании платежа | |
back_url | string | URL, куда перекинет пользователя после оплаты | |
cancel_url | string | URL, куда перекинет пользователя в случае отмены оплаты | |
lang | string | Язык виджета (ru-RU, en-EN) |
Параметры ответа:
Возвращает |
Объект виджета инвойса:
|
Пример ответа:
{ "success": true, "data": { "id": "523654", "final_amount": "133.23", "wallet": "TNVq3iEcaGWbbsR34MTdg1JMTxvYFU8Qir", "expire_at": "1677422490", "widget_url": "https://cryptoscan.one/payment/69908b3b-ac70-4652-9ca2-877e348a2dbe" } }
Просмотр Инвойса
Основная информация
Метод | GET invoice |
Пример URL | https://cryptoscan.one/api/v1/invoice/1 |
Параметры запроса
Имя параметра | Тип данных | Обязательно | Описание |
---|---|---|---|
id | integer | + | ID инвойса |
Параметры ответа:
Возвращает |
Объект инвойса:
|
Пример ответа:
{ "success": true, "data": { "id": 32, "wallet": "TBjkHCYgMb1ohJq77aovAyw4kCMtBEtMGN", "payer_wallet": null, "transaction_id": null, "final_amount": 10.54, "requested_amount": "10.54", "status": "completed", "client_reference_id": "287", "metadata": null, "created_at": 1678991717, "paid_at": null, "expire_at": 1678993517 } }
Поиск Инвойса
Основная информация
Метод | GET invoice |
Пример URL | https://cryptoscan.one/api/v1/invoice?query=123456789 |
Параметры запроса
Имя параметра | Тип данных | Обязательно | Описание |
---|---|---|---|
query | string | + |
Значение для поиска инвойсов.
Поиск указанного значения будет произведен в следующих полях:
|
Параметры ответа:
Возвращает |
Массив объектов инвойса:
|
Пример ответа:
{ "success": true, "data": { [ { "id": 36, "wallet": "TNVq3iEcaGWbbsR34MTdg1JMTxvYFU8Qir", "payer_wallet": "TNVq3iEcaGWbbsR34MTdg1JMTxvYFU8Qir", "transaction_id": "1234567890", "final_amount": 6.5, "requested_amount": "6.50000000", "status": "new", "client_reference_id": "539", "metadata": null, "created_at": 1679584937, "paid_at": 1679585937, "expire_at": 1679586737 } ] } }
Просмотр информации о пользователе (себе)
Информация
Метод | GET user |
Пример URL | https://cryptoscan.one/api/v1/user |
Параметры ответа:
Возвращает |
Объект данных о пользователе (себе):
|
Пример ответа:
{ "success": true, "data": { "id": 2, "status": "Active", "balance": 85.00, "currency": "USD" } }
Поддерживаемые валюты
Информация
Метод | GET currency-rate |
Пример URL | https://cryptoscan.one/api/v1/currency-rate |
Параметры ответа:
Возвращает |
Массив объектов валютных курсов:
Пример ответа:{ "success": true, "data": [ { "currency": "BYN", "rate": 2.52334, "status": "enabled" }, { "currency": "EUR", "rate": 0.93574, "status": "enabled" }, { "currency": "GBP", "rate": 0.80534, "status": "disabled" } ] } |
Проверка доступности валюты
Информация
Метод | GET currency-rate/status |
Пример URL | https://cryptoscan.one/api/v1/currency-rate/GBP/status |
Параметры запроса
Имя параметра | Тип данных | Обязательно | Описание |
---|---|---|---|
currency | string | + |
Код валюты в стандарте ISO 4217, которая будет проверена на доступность, например: EUR
|
Параметры ответа:
Возвращает |
Статус валютного курса:
|
Пример ответа:
{ "success": true, "data": { "status": "enabled", "rate": 0.93574 } }